diff --git a/README.md b/README.md index 7c1db4c0fe6598a3ae1181e39ce9103bfc4194a9..21606a1577f81d125a7f1f9bfbdcb89e67a12406 100644 --- a/README.md +++ b/README.md @@ -183,9 +183,9 @@ Series render styles include: `Round` and in the near future `Square`. 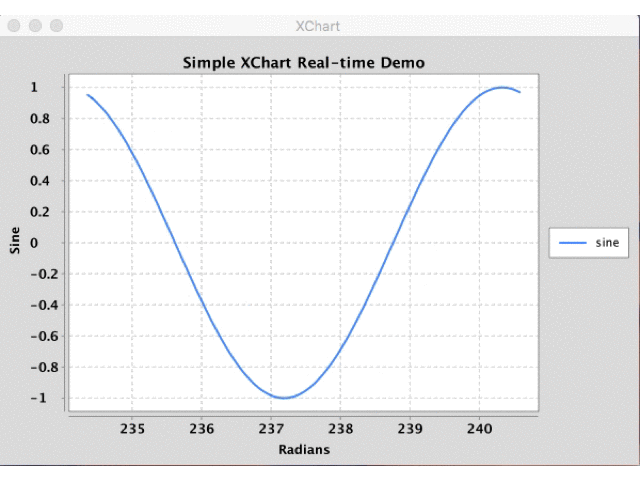 -Creating real-time charts is as simple as calling `updateXYSeries` for one or more series objects through the `XYChart` instance and triggering a redraw of the `JPanel` containing the chart. This works for all chart types including `XYChart`, `CategoryChart`, `BubbleChart` and `PieChart`, for which example source code can be found here: <https://github.com/timmolter/XChart/tree/develop/xchart-demo/src/main/java/org/knowm/xchart/demo/charts/realtime>. Examples demonstrate using the `SwingWrapper` with `repaintChart()` method as well as `XChartPanel` with `revalidate()` and `repaint()`. +Creating real-time charts is as simple as calling `updateXYSeries` for one or more series objects through the `XYChart` instance and triggering a redraw of the `JPanel` containing the chart. This works for all chart types including `XYChart`, `CategoryChart`, `BubbleChart` and `PieChart`, for which example source code can be found [here](https://github.com/timmolter/XChart/tree/develop/xchart-demo/src/main/java/org/knowm/xchart/demo/charts/realtime). Examples demonstrate using the `SwingWrapper` with `repaintChart()` method as well as `XChartPanel` with `revalidate()` and `repaint()`. -The following sample code used to generate the above real-time chart can be found [here](https://github.com/timmolter/XChart/blob/develop/xchart-demo/src/main/java/org/knowm/xchart/standalone/SimpleRealTime.java). +The following sample code used to generate the above real-time chart can be found [here](https://github.com/timmolter/XChart/blob/develop/xchart-demo/src/main/java/org/knowm/xchart/standalone/readme/SimpleRealTime.java). ```java public class SimpleRealTime { diff --git a/pom.xml b/pom.xml index 86fe7bbd78111f2d23ae92f221160e05549f6032..ea408883505f3f37a6e0916dfd245a8e72c98100 100644 --- a/pom.xml +++ b/pom.xml @@ -158,6 +158,7 @@ <version>2.10.4</version> <configuration> <additionalparam>-Xdoclint:none</additionalparam> + <excludePackageNames>org.knowm.xchart.internal.*</excludePackageNames> </configuration> </plugin> <!-- for deploying to Maven Central --> diff --git a/xchart-demo/src/main/java/org/knowm/xchart/standalone/readme/SwingWorkerRealTime.java b/xchart-demo/src/main/java/org/knowm/xchart/standalone/readme/SwingWorkerRealTime.java new file mode 100644 index 0000000000000000000000000000000000000000..8b3838e5f6cf091a9ea197cba5290fa8c861f3ac --- /dev/null +++ b/xchart-demo/src/main/java/org/knowm/xchart/standalone/readme/SwingWorkerRealTime.java @@ -0,0 +1,115 @@ +/** + * Copyright 2015-2016 Knowm Inc. (http://knowm.org) and contributors. + * Copyright 2011-2015 Xeiam LLC (http://xeiam.com) and contributors. + * + * Licensed under the Apache License, Version 2.0 (the "License"); + * you may not use this file except in compliance with the License. + * You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ +package org.knowm.xchart.standalone.readme; + +import java.util.LinkedList; +import java.util.List; + +import javax.swing.SwingWorker; + +import org.knowm.xchart.QuickChart; +import org.knowm.xchart.SwingWrapper; +import org.knowm.xchart.XYChart; + +/** + * Creates a real-time chart using SwingWorker + */ +public class SwingWorkerRealTime { + + HysteresisCaptureWorker hysteresisCaptureWorker; + SwingWrapper<XYChart> sw; + XYChart chart; + + public static void main(String[] args) throws Exception { + + SwingWorkerRealTime swingWorkerRealTime = new SwingWorkerRealTime(); + swingWorkerRealTime.go(); + } + + private void go() { + + // Create Chart + chart = QuickChart.getChart("SwingWorker XChart Real-time Demo", "Time", "Value", "randomWalk", new double[] { 0 }, new double[] { 0 }); + chart.getStyler().setLegendVisible(false); + chart.getStyler().setXAxisTicksVisible(false); + + // Show it + sw = new SwingWrapper<XYChart>(chart); + sw.displayChart(); + + hysteresisCaptureWorker = new HysteresisCaptureWorker(); + hysteresisCaptureWorker.execute(); + } + + private class HysteresisCaptureWorker extends SwingWorker<Boolean, double[]> { + + LinkedList<Double> fifo = new LinkedList<Double>(); + + public HysteresisCaptureWorker() { + + fifo.add(0.0); + } + + @Override + protected Boolean doInBackground() throws Exception { + + while (!isCancelled()) { + + fifo.add(fifo.get(fifo.size() - 1) + Math.random() - .5); + if (fifo.size() > 500) { + fifo.removeFirst(); + } + + double[] array = new double[fifo.size()]; + for (int i = 0; i < fifo.size(); i++) { + array[i] = fifo.get(i); + } + publish(array); + + try { + Thread.sleep(5); + } catch (InterruptedException e) { + // eat it. caught when interrupt is called + System.out.println("HysteresisCaptureWorker shut down."); + } + + } + + return true; + } + + @Override + protected void process(List<double[]> chunks) { + + System.out.println("number of chunks: " + chunks.size()); + + double[] mostRecentDataSet = chunks.get(chunks.size() - 1); + + chart.updateXYSeries("randomWalk", null, mostRecentDataSet, null); + sw.repaintChart(); + + long start = System.currentTimeMillis(); + long duration = System.currentTimeMillis() - start; + try { + Thread.sleep(40 - duration); // 40 ms ==> 25fps + // Thread.sleep(400 - duration); // 40 ms ==> 2.5fps + } catch (InterruptedException e) { + } + + } + } +}